Exceptions
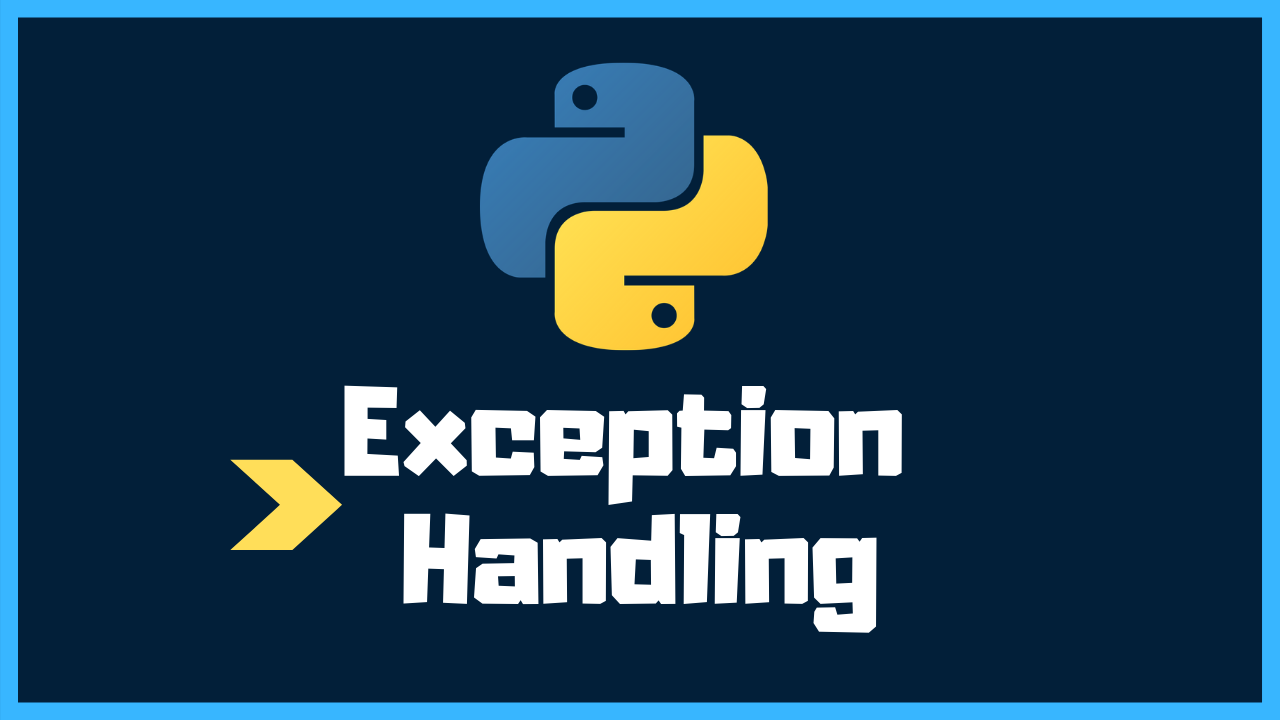
• Exception: error that occurs while a program is running
• Usually causes program to abruptly halt
• Traceback: error message that gives information regarding line numbers that caused the exception
• Indicates the type of exception and brief description of the error that caused exception to be raised
Exception handler: code that responds when exceptions are raised and prevents
program from crashing
In Python, written as try/except statement
General format:
try:
statements
except exceptionName:
statements
• Many exceptions can be prevented by careful coding
• Example: input validation
• Usually involve a simple decision construct
• Some exceptions cannot be avoided by careful coding
• Examples
• Trying to convert non-numeric string to an integer
• Trying to open for reading a file that doesn't exist
• If statement in try suite raises exception:
• Exception specified in except clause:
• Handler immediately following except clause executes
• Continue program after try/except statement
• Other exceptions:
• Program halts with traceback error message
• Trying to open for reading a file that doesn't exist
• If no exception is raised, handlers are skipped
HANDLING MULTIPLE EXCEPTIONS
• Often code in try suite can throw more than one type of exception
• Need to write except clause for each type of exception that
needs to be handled
• An except clause that does not list a specific exception will
handle any exception that is raised in the try suite
• Should always be last in a series of except clauses
• Program halts with traceback error message
• Trying to open for reading a file that doesn't exist
• If no exception is raised, handlers are skipped
DISPLAYING AN EXCEPTION'S DEFAULT ERROR MESSAGE
• Exception object: object created in memory when an exception is thrown
• Usually contains default error message pertaining to the exception
• An except clause that does not list a specific exception will
handle any exception that is raised in the try suite
• Can assign the exception object to a variable in an except clause
• Example: except ValueError as err:
• Can pass exception object variable to print function to display
the default error message
THE ELSE CLAUSE
• try/except statement may include an optional else clause, which
appears after all the except clauses
• Aligned with try and except clauses
• Syntax similar to else clause in decision structure
•Else suite: block of statements executed after statements in try
suite, only if no exceptions were raised
• If exception was raised, the else suite is skipped
THE FINALLY CLAUSE
• try/except statement may include an optional finally clause, which appears after all
the except clauses
• Aligned with try and except clauses
• General format:
finally:
statements
• Finally suite: block of statements after the finally clause
• Execute whether an exception occurs or not
• Purpose is to perform cleanup before exiting
WHAT IF AN EXCEPTION IS NOT HANDLED?
Two ways for exception to go unhandled:
• No except clause specifying exception of the right type
• Exception raised outside a try suite
In both cases, exception will cause the program to halt
• Python documentation provides information about
exceptions that can be raised by different functions