DICTIONARIES
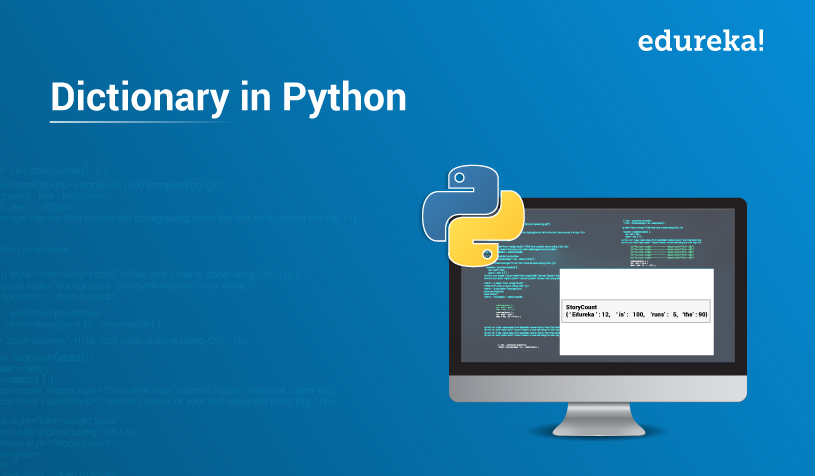
• Dictionary: object that stores a collection of data
• Each element consists of a key and a value
• Often referred to as mapping of key to value
• Key must be an immutable object
• To retrieve a specific value, use the key associated with it
• Format for creating a dictionary
dictionary = {key1:val1, key2:val2}
ADD AND DELETE DICTIONARY
• Dictionaries are mutable objects
• To add a new key-value pair: dictionary[key] = value
• If key exists in the dictionary, the value associated
with it will be changed
• To delete a key-value pair: del dictionary[key]>
• If key is not in the dictionary, KeyError exception is
raised
DICTIONARY NUMBER OF ELEMENTS AND MIXING DATA TYPES
• len function: used to obtain number of elements in a dictionary
• Keys must be immutable objects, but associated values can be any type of object
• One dictionary can include keys of several different
immutable types
• Values stored in a single dictionary can be of different types
CREATING AN EMPTY DICTIONARY AND USING FOR LOOP
• To create an empty dictionary:
• Use {}
• Use built-in function dict()
• Elements can be added to the dictionary as program
executes
• Use a for loop to iterate over a dictionary
• General format: for key in dictionary:
for key in dictionary:
SOME DICTIONARY METHODS
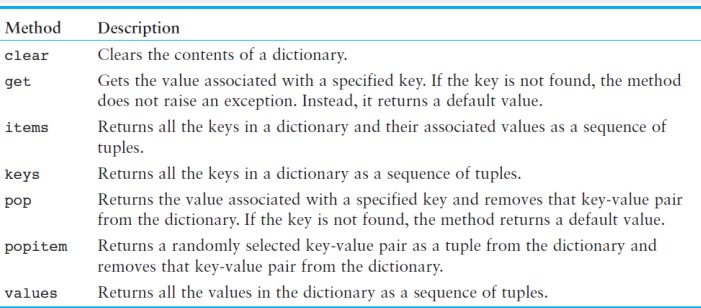