Set Methods and Useful Built-in Functions
Set
• Set: object that stores a collection of data in same
way as mathematical set
• All items must be unique
• Set is unordered
• Elements can be of different data types
• len function: returns the number of elements in the set
• Sets are mutable objects
• add method: adds an element to a set
• update method: adds a group of elements to a set
• Argument must be a sequence containing iterable
elements, and each of the elements is added to the set
• remove and discard methods: remove the specified item from the set
• The item that should be removed is passed to both methods as
an argument
• Behave differently when the specified item is not found in the set
• remove method raises a KeyError exception
• discard method does not raise an exception
• clear method: clears all the elements of the set
FINDING THE UNION OF SETS
• Union of two sets: a set that contains all the elements of both sets• To find the union of two sets:
• Use the union method
• Format: set1.union(set2)
• Use the | operator
• Format: set1 | set2
• Both techniques return a new set which contains the union of both sets
FINDING THE INTERSECTION OF SETS
• Intersection of two sets: a set that contains only the elements
found in both sets
• To find the intersection of two sets:
• Use the intersection method
• Format: set1.intersection(set2)
• Use the & operator
• Format: set1 & set2
• Both techniques return a new set which contains the intersection of
both sets
FINDING THE SYMMETRIC DIFFERENCE OF SETS
• Symmetric difference of two sets: a set that contains
the elements that are not shared by the two sets
• To find the symmetric difference of two sets:
• Use the symmetric_difference method
• Format: set1.symmetric_difference(set2)
• Use the ^ operator
• Format: set1 ^ set2
FINDING SUBSETS AND SUPERSETS
• Set A is subset of set B if all the elements in set A are included in set B
• To determine whether set A is subset of set B
• Use the issubset method
• Format: setA.issubset(setB)
• Use the <= operator
• Format: setA <= setB
• Set A is superset of set B if it contains all the elements of set B
• To determine whether set A is superset of set B
• Use the issuperset method
• Format: setA.issuperset(setB)
• Use the >= operator
• Format: setA >= setB
SET OPERATION
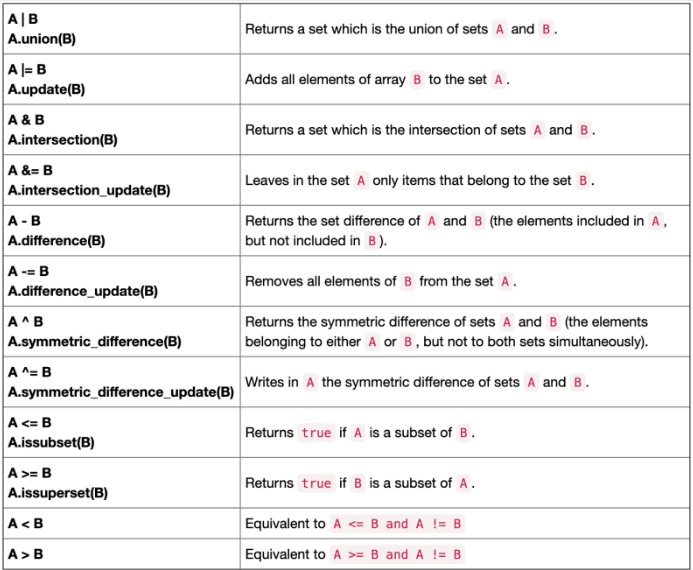